jQuery Ancestors in Hindi
jQuery Traversing में ancestors के जरिये किसी element की parent, grant-parent, great-grant-parent और ऊपर के ancestors को target किया जा सकता है |
ancestors methods कई methods का समारोह है और वो है:
- parent
- parents
- parentsUntil
- offsetParent
- closest
parent()
parent() method के जरिये किसी element के immediate parent को select किया जा सकता है | ये method, DOM tree में सिर्फ एक ही top level तक traverse करता है |
उदाहरण:
<!DOCTYPE html> <html> <head> <style> .grant-parent{ border: 2px solid gray; background-color: rgb(247, 222, 222); padding: 5px; margin: 10px; } .parent{ border: 2px solid blue; background-color: sandybrown; padding: 5px; margin: 10px; } .child{ display: inline-block; border: 2px solid gray; background-color: rgb(207, 241, 155); padding: 5px; margin: 10px; width:100px; height: 50px;; } </style> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"> </script> <script> $(document).ready(function(){ $("#child1").parent().css({ "background-color": "yellow" }); }); </script> </head> <body> <div class="grant-parent" style="width: 500px;"> <h2>grant parent</h2> <div class="parent"> <h2>parent</h2> <div class="child" id="child1">child1</div> <div class="child" id="child2">child2</div> </div> </div> </body> </html>
output
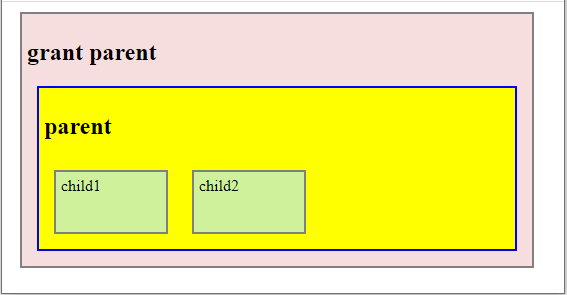
उदाहरण में देखिए child1 का जो immediate parent है सिर्फ उसीका background-color बदला है |
parents()
इस method से element के जितने भी ancestors हैं parent, grant-parent, great-grant parent सारे एक साथ select हो जातें हैं |
उदाहरण:
<!DOCTYPE html> <html> <head> <style> .grant-parent{ border: 2px solid gray; background-color: rgb(247, 222, 222); padding: 5px; margin: 10px; } .parent{ border: 2px solid blue; background-color: sandybrown; padding: 5px; margin: 10px; } .child{ display: inline-block; border: 2px solid gray; background-color: rgb(207, 241, 155); padding: 5px; margin: 10px; width:100px; height: 50px;; } </style> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"> </script> <script> $(document).ready(function(){ $("#child1").parents().css({ "background-color": "aliceblue" }); }); </script> </head> <body> <div class="grant-parent" style="width: 500px;"> <h2>grant parent</h2> <div class="parent"> <h2>parent</h2> <div class="child" id="child1">child1</div> <div class="child" id="child2">child2</div> </div> </div> </body> </html>
output
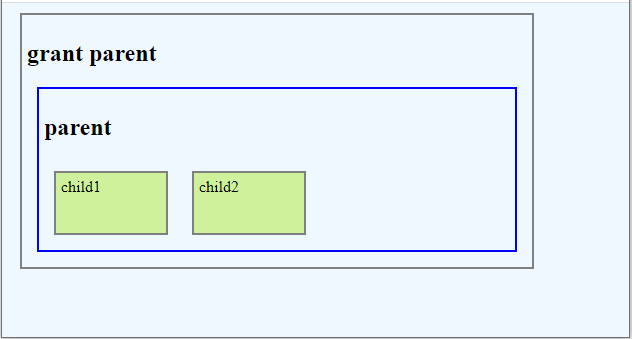
output में देखिये child1 का सभी parents का background-color अब बदल गया है | ये body tag का भी background-color बदल दिया है क्यूँ की body tag सभी elements का root parent होता है |
parents() method में condition लगाके हम किसी specific parent को भी target कर सकतें हैं | जैसे की child1 की grant-parent को target करना हो तो उदाहरण में देखिये:
उदाहरण:
<script> $(document).ready(function(){ $("#child1").parents('.grant-parent').css({ "background-color": "aliceblue" }); }); </script>
output
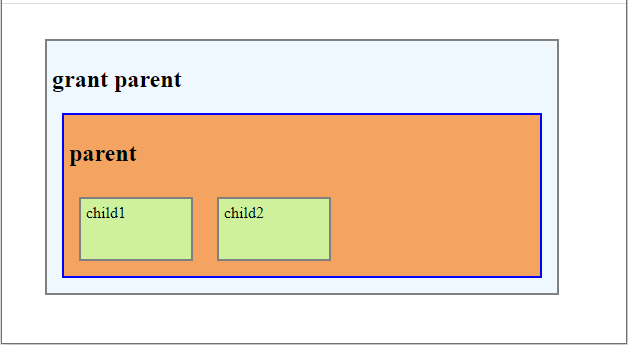
output में देखिए हमने parents() method में condition लगाकर सिर्फ grant-parent की background-color बदली है |
parentsUntil()
parentsUntil() method के जरिये selector के कोनसे parents तक target करना है वो condition लगा सकतें हैं |
Syntax: $(selector).parentsUntil(stop);
stop parameter बताता है की कहाँ तक target करना है |
उदाहरण:
<!DOCTYPE html> <html> <head> <style> .great-grant-parent{ border: 2px solid gray; background-color: rgb(247, 222, 222); padding: 10px; margin: 35px; } .grant-parent{ border: 2px solid rgb(48, 48, 78); background-color: rgb(166, 240, 203); padding: 10px; margin: 10px; } .parent{ border: 2px solid blue; background-color: sandybrown; padding: 10px; margin: 10px; } .child{ display: inline-block; border: 2px solid gray; background-color: rgb(207, 241, 155); padding: 5px; margin: 10px; width:100px; height: 50px;; } </style> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"> </script> <script> $(document).ready(function(){ $("#child1").parentsUntil('.great-grant-parent').css({ "background-color": "yellow" }); }); </script> </head> <body> <div class="great-grant-parent" style="width: 600px;"> <h2>great grant parent</h2> <div class="grant-parent" id="grant-parent"> <h2>grant parent</h2> <div class="parent"> <h2>parent</h2> <div class="child" id="child1">child1</div> <div class="child" id="child2">child2</div> </div> </div> </div> </body> </html>
output
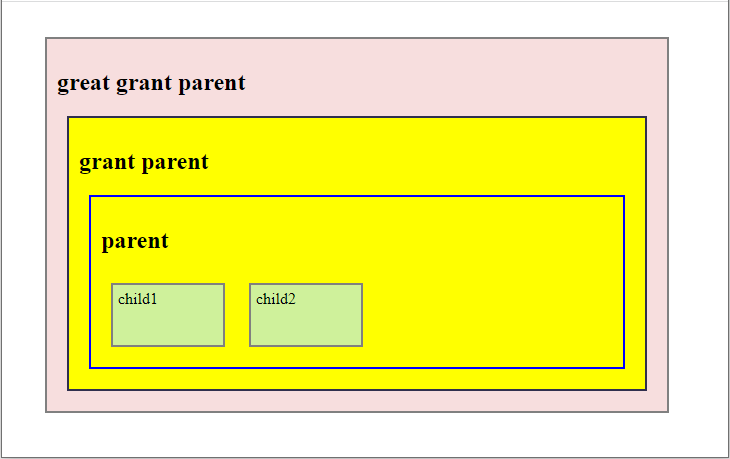
उदाहरण में देखिये हमने stop parameter में great-grant-parent डाला था इसीलिए child1 से लेकर great-grant-parent तक जितने भी parents थे उसे ये target कर लिया |
offsetParent()
offsetParent() method उन parent elements को target करता है जिसमें position property लगी होती है |
उदाहरण:
<!DOCTYPE html> <html> <head> <style> .great-grant-parent{ border: 2px solid gray; background-color: rgb(247, 222, 222); padding: 10px; margin: 35px; } .grant-parent{ border: 2px solid rgb(48, 48, 78); background-color: rgb(166, 240, 203); padding: 10px; margin: 10px; } .parent{ border: 2px solid blue; background-color: rgb(229, 247, 127); padding: 10px; margin: 10px; } .child{ display: inline-block; border: 2px solid gray; background-color: rgb(224, 250, 185); padding: 5px; margin: 10px; width:100px; height: 50px;; } </style> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"> </script> <script> $(document).ready(function(){ $("#child1").offsetParent().css({"background-color": "blue"}); }); </script> </head> <body> <div class="great-grant-parent" style="width: 600px;"> <h2>great grant parent</h2> <div class="grant-parent" id="grant-parent" style="position:relative;"> <h2>grant parent</h2> <div class="parent"> <h2>parent</h2> <div class="child" id="child1">child1</div> <div class="child" id="child2">child2</div> </div> </div> </div> </body> </html>
output
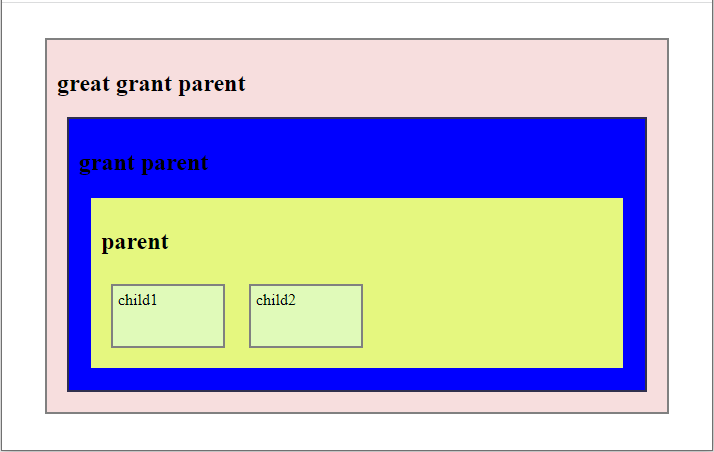
उदाहरण में देखिये grant-parent में position property लगा था इसीलिए उसीकी background-color बदला |
नॉटपॉइंट : offsetParent method उस पहले parent को select करता जिसमें position property लगी होती है |
closest()
ये condition based method है, इसमें pass किया हुआ parameter के हिसाब से जो भी नजदीकी parent मिलता है उसे select करता है |
पर ये DOM tree में current element यानि खुदसे सुरुआत करता है |
Syntax: $(selector).closest(filter);
उदाहरण:
<script> $(document).ready(function(){ $("#child1").closest('div').css({"background-color": "blue"}); }); </script>